Contributing
Guide for new contributors
Welcome 🥳
Reor is aiming to be the world's first self-organizing knowledge base. That's a big goal and we need all the help we can get! This is a guide for new contributors to get setup and understand the architecture.
Setting up
1. Fork the repo and clone it:
Head here and fork the repo. Then clone your fork:
git clone https://github.com/YourUserName/reor.git
cd reor
2. Install dependencies:
Make sure you have nodejs installed. Run:
npm install
3. Run for dev:
npm run dev
(It won't be able to start if you already have Reor running. You'll need to close the app first)
4. Build:
npm run build
5. Run the linter:
npm run lint:fix && npm run type-check
6. Open a PR:
git checkout -b new-branch
git add .
git commit -m "Awesome changes I made"
git push origin new-branch
Then open a PR. It should prompt you to open a PR when you push the branch anyways :)
Bounties
Some points on how bounties work in Reor:
- If someone is already assigned to an issue, please do not attempt it or try to submit a bounty!
- To avoid duplicate work, please wait until you are assigned to an issue before starting work :)
- You are more likely to get assigned to an issue if you write a comment about how your proposal.
- If you work on a bounty and something isn't clear, please ask questions - that way things get resolved without wasting work!
- Submitting an issue or a bug is not just a great way to contribute but we are more than likely to assign a bounty to it and will give you priority to work on it.
Architecture Overview
Reor is an Electron app built with React and TypeScript. The app is split into two main parts:
- The main process is a Node.js process responsible for things like making LLM calls, managing the vector database and talking to the filesystem.
- The renderer process is basically a React app with all the things you see in the app.
The main and renderer processes can't directly call eachother. They communicate via message passing. The renderer process invokes a function (specified in preload) which sends a message to the main process.
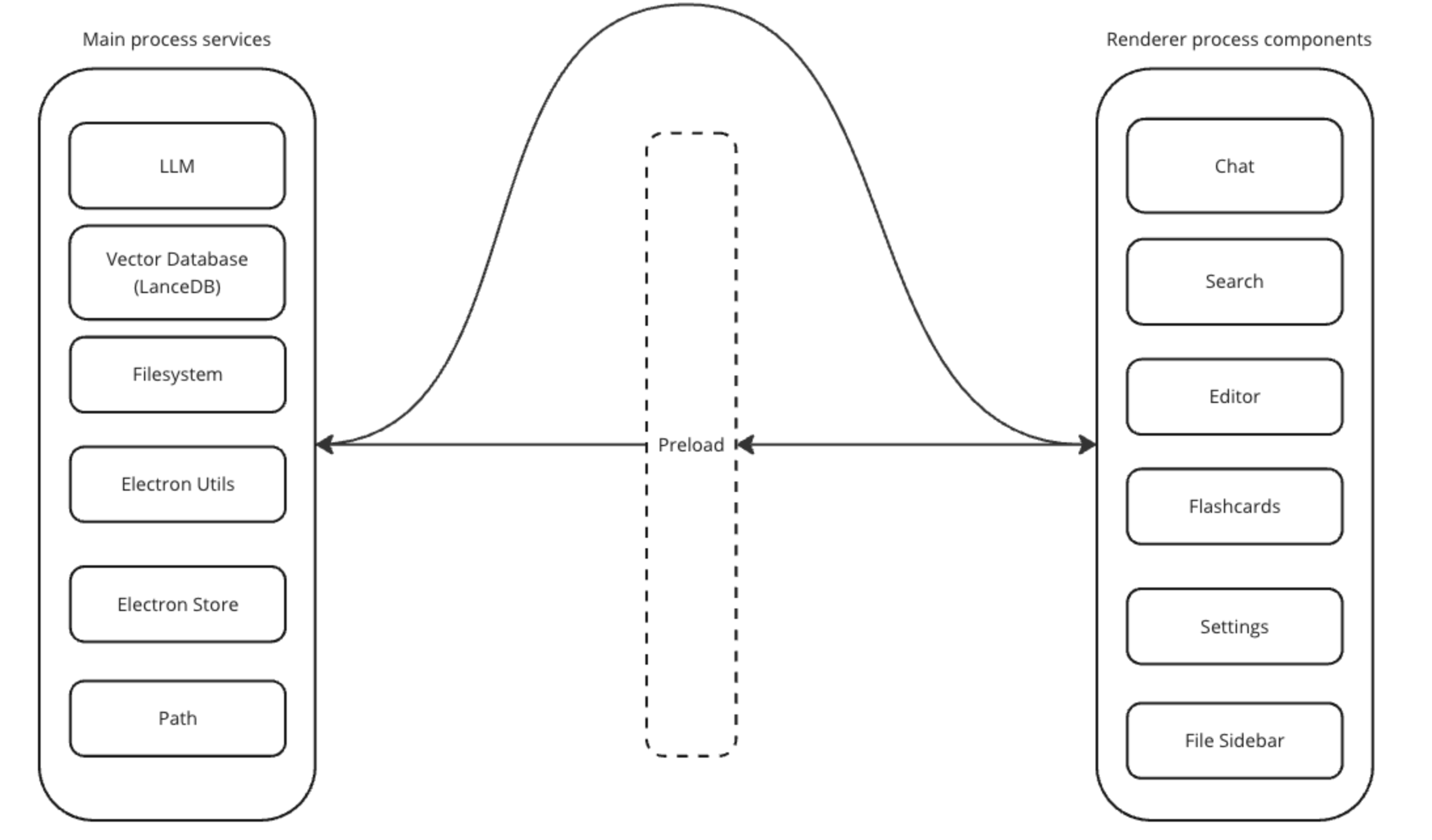
The main process is in the electron
folder and the renderer process is in the src
folder. The preload file is in the electron/preload/index.ts
.
Main process services
LLM
- All functions for calling OpenAI, Anthropic and Ollama LLMs.
- Manages local LLMs via Ollama including downloads. (We package the Ollama binary with Reor).
- Has streaming endpoints which sends chunks back to the renderer using "openAITokenStream" and "anthropicTokenStream" channels.
Vector database
- Manages the LanceDB vector database.
- Provides search and indexing functionality.
- Manages local embedding models through Transformers.js.
Filesystem
- Pretty straightforwardly provides functions to do things like write and read files from disk.
- Processes files into
FileInfo
objects which are basically just file metadata. - Provides watcher functionality for watching the user's vault directory and sending updates to the renderer.
Electron utils
- Miscellaneous functions for things like showing context windows, opening links in browsers and opening new Reor windows.
Electron store
- A wrapper around the Electron store package for storing user settings and preferences.
- Currently also stores chat history (though this will be moved to the user's vault soon!)
Path
- Utils for using node.js's path module.
- Basically helpful for things like joining paths and getting file extensions (where we don't want to make assumptions about the OS)